Developer Guide
Projact - Developer Guide
Projact is a project and contact management app that helps NUS computing students to organise their fellow computing students’ contacts and their teams’ meeting links and tasks.
This developer guide provides information that helps you to get started as a contributor to Projact.
Table of Contents
- Setting up
- Design
- Implementation
- Documentation, logging, testing, configuration, dev-ops
- Appendix
Setting up, getting started
Refer to the guide Setting up and getting started.
Design
Architecture
The Architecture Diagram given above explains the high-level design of the App. Given below is a quick overview of each component.

.puml
files used to create diagrams in this document can be found in the diagrams folder. Refer to the PlantUML Tutorial at se-edu/guides to learn how to create and edit diagrams.
Main
has two classes called Main
and MainApp
. It is responsible for,
- At app launch: Initializes the components in the correct sequence, and connects them up with each other.
- At shut down: Shuts down the components and invokes cleanup methods where necessary.
Commons
represents a collection of classes used by multiple other components.
The rest of the App consists of four components.
-
UI
: The UI of the App. -
Logic
: The command executor. -
Model
: Holds the data of the App in memory. -
Storage
: Reads data from, and writes data to, the hard disk.
Each of the four components,
- defines its API in an
interface
with the same name as the Component. - exposes its functionality using a concrete
{Component Name}Manager
class (which implements the corresponding APIinterface
mentioned in the previous point.
For example, the Logic
component (see the class diagram given below) defines its API in the Logic.java
interface and exposes its functionality using the LogicManager.java
class which implements the Logic
interface.
How the architecture components interact with each other
The Sequence Diagram below shows how the components interact with each other for the scenario where the user issues the command delete 1
.
The sections below give more details of each component.
UI component
API :
Ui.java
The UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
, PersonListPanel
, StatusBarFooter
etc. All these, including the MainWindow
, inherit from the abstract UiPart
class.
The UI
component uses JavaFx UI framework. The layout of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
The UI
component,
- Executes user commands using the
Logic
component. - Listens for changes to
Model
data so that the UI can be updated with the modified data.
Logic component
API :
Logic.java
-
Logic
uses theProjactParser
class to parse the user command. - This results in a
Command
object which is executed by theLogicManager
. - The command execution can affect the
Model
(e.g. adding a person). - The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUi
. - In addition, the
CommandResult
object can also instruct theUi
to perform certain actions, such as displaying help to the user.
Given below is the Sequence Diagram for interactions within the Logic
component for the execute("delete 1")
API call.

DeleteCommandParser
should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of diagram.
Model component
API : Model.java
The Model
,
- stores a
UserPref
object that represents the user’s preferences. - stores the projact data.
- exposes unmodifiable
ObservableList<Person>
andObservableList<Tag>
that can be ‘observed’ e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. - does not depend on any of the other three components.

Tag
list in the Projact
, and its TagName
is referenced by Person
. This allows Projact
to only require one Tag
object per unique Tag
, instead of each Person
needing their own Tag
object.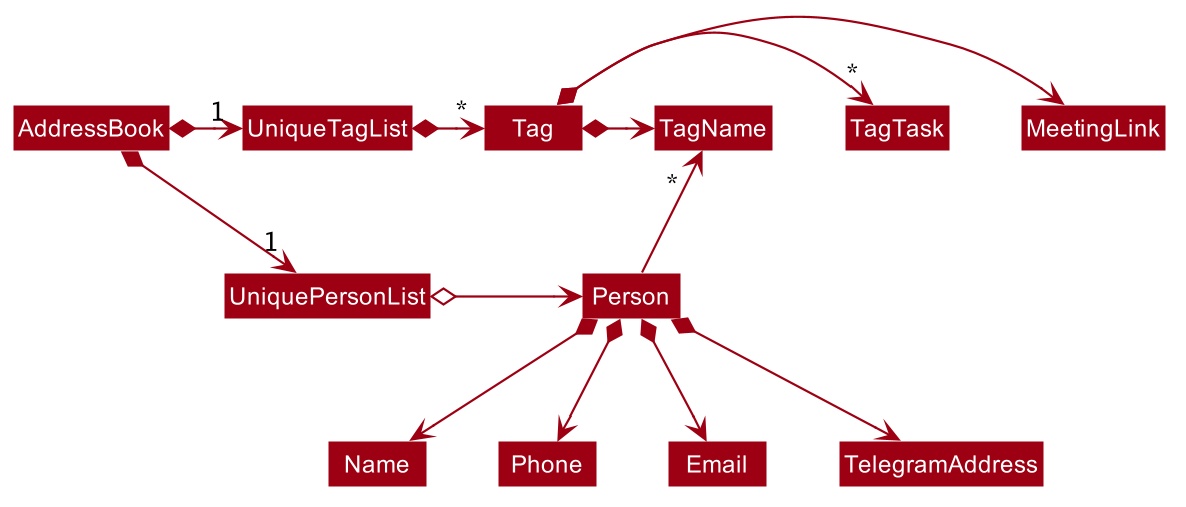
Storage component
API : Storage.java
The Storage
component,
- can save
UserPref
objects in json format and read it back. - can save projact data in json format and read it back.
Common classes
Classes used by multiple components are in the seedu.addressbook.commons
package.
Implementation
Contact features
Sort command
The sort
command allows a user to sort the current person list in alphanumerical order.
- How is SortContactCommand executed
- The command is passed into
LogicManager
-
LogicManager
calls parseCommand method ofProjactParser
. -
ProjactParser
identifies and returnsSortCommand
. -
LogicManager
executesSortCommand
, which updates the sorted person list by comparing the personNames usingPersonNameComparator
inModel
.
- The command is passed into
The diagram below shows a sample interaction of SortCommand.
- Why is it implemented that way?
- The overall implementation flow of the
sort
command is similar to thefind
command in the original AB3 but instead of using Filtered List and Predicate, Sorted List and Comparator are used to sort the list.
- The overall implementation flow of the
Tag features
TagAdd command
The tagadd
command allows a user to add a new tag to the tag list. The tag added will not have any contacts under it initially.
- How is TagAddCommand executed
- The command is passed into
LogicManager
. -
LogicManager
calls the parseCommand method ofProjactParser
. -
ProjactParser
identifies the commandWord, which in this case is ‘tagadd’ and the arguments. -
ProjactParser
calls the parse method ofTagAddCommandParser
, which parses the argument, creates a newTag
object, and returns a newTagAddCommand
with the newTag
object used as an argument. - The
LogicManager
then calls the execute method of theTagAddCommand
, which calls the addTag method ofModel
.
- The command is passed into
The diagram below shows a sample interaction of TagAddCommand
.
- Why is it implemented that way:
- The command is implemented to be as similar as possible to the current command classes, so that there will be minimal changes to the overall design of the product. Most new classes added to accommodate the TagAddCommand will also be largely similar to classes implemented in AB3.
TagEdit command
The tagedit
command allows a user to edit the tag name of a tag in the list.
- How is TagEditCommand executed
- The command is passed in to
LogicManager
. -
LogicManager
calls the parseCommand method ofProjactParser
. -
ProjactParser
identifies the commandWord, which in this case is ‘tagedit’ and the arguments. -
ProjactParser
calls the parse method ofTagEditCommandParser
, which parses the argument, creates a newIndex
object and a newEditTagDescriptor
object, and returns a newTagEditCommand
with those objects used as arguments. - The
LogicManager
then calls the execute method of theTagEditCommand
, which create a newTag
object with the edited field and replaces the currentTag
object at the specified index inFilteredTagList
. -
FilteredTagList
is updated with the editedTag
object and will reflect the changes in theModel
.
- The command is passed in to
The diagram below shows a sample interaction of TagEditCommand
.
- Why is it implemented that way:
- The implementation of the TagEdit command is very similar to the Edit command so that we can reuse the previous code.
- For example, by making the commandWord ‘tagedit’ instead of ‘tag edit’, we are able to make use of
ProjectParser
instead of creating a different parser just to identify tag commands.
TagList command
The taglist
command allows a user to display all the tags in the tag list currently.
- How is TagListCommand executed
- The command is passed into
LogicManager
. -
LogicManager
calls parseCommand method ofProjactParser
. -
ProjactParser
identifies and returnsTagListCommand
. -
LogicManager
executesTagListCommand
, which sets isTagList parameter inCommandResult
to true. -
MainWindow
detectsCommandResult
isTagList() returns true and hence invokes the showTagList() method.
- The command is passed into
The diagram below shows a sample interaction of TagListCommand
.
- Why is it implemented that way:
- The command is implemented to be as similar as possible to the current command classes, so that there will be minimal changes to the overall design of the product. Most new classes added to accommodate the
TagListCommand
will also be largely similar to classes implemented in AB3.
- The command is implemented to be as similar as possible to the current command classes, so that there will be minimal changes to the overall design of the product. Most new classes added to accommodate the
TagFind command
The tagfind
command allows a user to display all tags which contains at least one of the specified keywords.
- How is TagFindCommand executed
- The command is passed in to
LogicManager
. -
LogicManager
calls the parseCommand method ofProjactParser
. -
ProjactParser
identifies the commandWord, which in this case is ‘tagfind’ and the arguments. -
ProjactParser
calls the parse method ofTagFindCommandParser
, which parses the argument, creates a newTagNameContainsKeywordsPredicate
object with the keywords, and returns a newTagFindCommand
with the newTagNameContainsKeywordsPredicate
object used as an argument. - The
LogicManager
then calls the execute method of theTagFindCommand
, which calls the updateFilteredTagList method ofModel
. This method takes in aPredicate<Tag>
and filters theTagList
by the supplied predicate.
- The command is passed in to
The diagram below shows a sample interaction of TagFindCommand
.
- Why is it implemented that way:
- The command is implemented to be as similar as possible to the current command classes, so that there will be minimal changes to the overall design of the product. Most new classes added to accommodate the
TagFindCommand
will also be largely similar to classes implemented in AB3.
- The command is implemented to be as similar as possible to the current command classes, so that there will be minimal changes to the overall design of the product. Most new classes added to accommodate the
TagSort command
The tagsort
command allows a user to sort the tag list in alphanumerical order.
The implementation and interaction of the TagSortCommand
is similar to SortContactCommand
but it uses TagNameComaparator
instead of PersonNameComparator
.
- Why is it implemented that way:
- The TagSort and SortContact commands share similar functions with the former sorting the tag list and the latter sorting the person list. Hence, a similar set of methods are for this feature to ensure the application can work smoothly.
TagDelete command
The tagdelete
command allows a user to delete a tag permanently. This feature will result in the removal of the tag from the tag list and from any contact with said tag.
- How is TagDeleteCommand executed
- The command is passed in to
LogicManager
. -
LogicManager
calls the parseCommand method ofProjactParser
. -
ProjactParser
identifies the commandWord, which in this case is ‘tagdelete’ and the arguments. -
ProjactParser
calls the parse method ofTagDeleteCommandParser
, which parses the argument, creates a newIndex
object with the parsed user input, and returns a newTagDeleteCommand
with the newIndex
object used as an argument. - The
LogicManager
then calls the execute method of theTagDeleteCommand
, which retrieves the most updated tag list from theModelManager
. From this list, the tag to be deleted is retrieved by its index. Then, theModelManager
will go on to remove all instances of the tag.
- The command is passed in to
The diagram below shows a sample interaction of TagDeleteCommand
.
- Why is it implemented that way:
- The command is implemented to be as similar as possible to the current command classes, so that there will be minimal changes to the overall design of the product. Most new classes added to accommodate the
TagDeleteCommand
will also be largely similar to classes implemented in AB3.
- The command is implemented to be as similar as possible to the current command classes, so that there will be minimal changes to the overall design of the product. Most new classes added to accommodate the
Link Features
LinkAdd command
The linkadd
command allows a user to add a meeting link to a specified tag.
- How is LinkAddCommand executed
- The command is passed into
LogicManager
. -
LogicManager
calls the parseCommand method ofProjactParser
. -
ProjactParser
identifies the commandWord, which in this case is ‘linkadd’ and the arguments. -
ProjactParser
calls the parse method ofLinkAddCommandParser
, which parses the argument, creates newOptional<MeetingLink>
andIndex
objects, and returns a newLinkAddCommand
with the newOptional<MeetingLink>
andIndex
objects used as arguments. - The
LogicManager
then calls the execute method of theLinkAddCommand
, which creates a newTag
object with theOptional<MeetingLink>
object, and replaces the currentTag
object at the specified index inFilteredTagList
. -
FilteredTagList
is updated with the editedTag
object and will reflect the changes in theModel
.
- The command is passed into
The diagram below shows a sample interaction of LinkAddCommand
.
- Why is it implemented this way:
- The command is implemented to be as similar as possible to the current command classes, so that there will be minimal changes to the overall design of the product. Most new classes added to accommodate the
LinkAddCommand
will also be largely similar to classes implemented in AB3.
- The command is implemented to be as similar as possible to the current command classes, so that there will be minimal changes to the overall design of the product. Most new classes added to accommodate the
LinkDelete command
The linkdelete
command allows a user to delete the meeting link from a tag permanently.
- How is LinkDeleteCommand executed:
- The command is passed in to
LogicManager
. -
LogicManager
calls the parseCommand method ofProjactParser
. -
ProjactParser
identifies the command word, which in this case is ‘linkdelete’ and the arguments. -
ProjactParser
calls the parse method ofLinkDeleteCommandParser
, which parses the argument, creates a newIndex
object with the parsed user input, and returns a newLinkDeleteCommand
with the newIndex
object used as an argument. - The
LogicManager
then calls the execute method of theLinkDeleteCommand
, which retrieves the most updated tag list from theModelManager
. From this list, the tag that will has its meeting link being removed will be retrieved. A new tag will be created with an empty meeting link using Optional class and replaced the old tag retrieved. - Then, the
ModelManager
will update the tag list.
- The command is passed in to
The diagram below shows a sample interaction of LinkDeleteCommand
.
- Why is it implemented that way:
- The command is implemented to be as similar as possible to the current command classes, so that there will be minimal changes to the overall design of the product. Most new classes added to accommodate the
LinkDeleteCommand
will also be largely similar to classes implemented in AB3.
- The command is implemented to be as similar as possible to the current command classes, so that there will be minimal changes to the overall design of the product. Most new classes added to accommodate the
Task Features
TaskAdd command
The taskadd
command allows the user to add a task to a tag.
- How is TaskAddCommand executed:
- The command is passed into
LogicManager
. -
LogicManager
calls the parseCommand method ofProjactParser
. -
ProjactParser
identifies the commandWord, which in this case is ‘taskadd’ and the arguments. -
ProjactParser
calls the parse method ofTaskAddCommandParser
, which parses the argument, creates a neweditTagDescriptor
object, and returns a newTaskAddCommand
with the neweditTagDescriptor
object used as an argument. - The
LogicManager
then calls the execute method of theTaskAddCommand
, which calls the setTag method ofModel
.
- The command is passed into
The diagram below shows a sample interaction of TaskAddCommand
.
- Why is it implemented that way:
- The command is implemented to be as similar as possible to the current command classes, so that there will be minimal changes to the overall design of the product. Most new classes added to accommodate the
TaskAddCommand
will also be largely similar to classes implemented in AB3.
- The command is implemented to be as similar as possible to the current command classes, so that there will be minimal changes to the overall design of the product. Most new classes added to accommodate the
TaskDone command
The taskdone
command allows the user to mark a task under a tag as done.
- How is TaskDoneCommand executed:
- The command is passed in to
LogicManager
. -
LogicManager
calls the parseCommand method ofProjactParser
. -
ProjactParser
identifies the command word, which in this case is ‘taskdone’ and the arguments. -
ProjactParser
calls the parse method ofTaskDoneCommandParser
, which parses the arguments, creates twoIndex
objects, the first one to be the tag Index and the second one is the task Index and returns a newTaskDoneCommand
with twoIndex
objects pass in as parameters. - The
LogicManager
then calls the execute method of theTaskDoneCommand
, which retrieves the tag from the taglist based on the tag Index. From the tag, the task list will be retrieved to get the targeted task using the task Index. The targeted task will be marked done and a new tag with the modified task list will be created and replaced the old Tag. - Then, the
ModelManager
will update the tag list.
- The command is passed in to
The diagram below shows a sample interaction of TaskDoneCommand
.
- Why is it implemented that way:
- The command is implemented such that there is no need for any prefixes. This helps to shorten the command and reduces the time required to mark a task as done.
- The second index uses an alphabetical index instead of a numerical one. This avoids confusion between the index of the tag and the index of the task.
TaskDelete command
The taskdelete
command allows the user to delete a task from a tag.
- How is TaskDeleteCommand executed:
- The command is passed into
LogicManager
. -
LogicManager
calls the parseCommand method ofProjactParser
. -
ProjactParser
identifies the commandWord, which in this case istaskdone
and the arguments. -
ProjactParser
calls the parse method ofTaskDeleteCommandParser
, which parses the argument, cand returns a newTaskDeleteCommand
. - The
LogicManager
then calls the execute method of theTaskDeleteCommand
, which calls the setTag method ofModel
.
- The command is passed into
- Why is it implemented that way:
- The design consideration on the indices for the
TaskDeleteCommand
is similar toTaskDoneCommand
.
- The design consideration on the indices for the
The diagram below shows a sample interaction of TaskDeleteCommand
.
TaskClear command
The taskclear
command allows the user to clear all completed tasks under a tag.
- How is TaskClearCommand executed:
- The command is passed in to
LogicManager
. -
LogicManager
calls the parseCommand method ofProjactParser
. -
ProjactParser
identifies the command word, which in this case is ‘taskclear’ and the arguments. -
ProjactParser
calls the parse method ofTaskClearCommandParser
, which parses the argument, creates a newIndex
object and returns a newTaskClearCommand
with the newIndex
object as an argument. - The
LogicManager
then calls the execute method of theTaskClearCommand
, which retrieves theTag
object at the specified index inFilteredTagList
. From theTag
, a list of its uncompleted tasks,List<TagTask>
, is obtained. - A new
Tag
object is created with the newList<TagTask>
and replaces the currentTag
object at the specified index inFilteredTagList
. -
FilteredTagList
is then updated to reflect the changes inModel
.
- The command is passed in to
The diagram below shows a sample interaction of TaskDoneCommand
.
- Why is it implemented that way:
- The command is implemented to be similar to the current command classes, so that there will be minimal changes to the overall design of the product.
- An alternative implementation would be for
TaskClearCommand
to not take in an index and just delete all completed tasks from every tag.- However, the user may want to keep certain tags with completed tasks for tracking purposes.
- Furthermore, this implementation will result in a significantly long execution time for the
TaskClearCommand
as the number of tags and tasks increase.
Documentation, logging, testing, configuration, dev-ops
Appendix
A: Product Scope
Target user profile: Computing Student
- has a need to manage a significant number of computing student contacts
- prefer desktop apps over other types
- can type fast
- is reasonably comfortable using CLI apps
- wants to group contacts based on the different modules
- has a need to store different url links for each module in a single accessible location
- wants to keep track of tasks
Value propositions:
- Manages contacts faster than a typical mouse/GUI driven app.
- Sorts contacts based on the different modules.
- Allows convenient search for project mates / friends taking the same module.
- Allows convenient search for the link for team meeting.
- Has a to-do list to keep track of task progress.
B: User Stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a … | I want to … | So that I can… |
---|---|---|---|
* * * |
new user | see usage instructions | refer to instructions when I forget how to use the application. |
* * * |
new user | see sample contacts and tags | have a brief idea on what the app will look like when it is being used |
* * * |
user | add a new contact | |
* * * |
user | delete a contact | remove contacts that I no longer need |
* * * |
user | find a contact by name | locate details of persons without having to go through the entire list |
* * * |
user | save a telegram handle to its respective contact | find the telegram chat with that person quickly |
* * * |
user | create a tag | find or sort the contacts based on tags later on. |
* * * |
user | delete a tag | remove tags that are accidentally added or no longer required |
* * * |
user | find a tag by name | locate details of the tag without having to go through the entire list |
* * * |
user | list all tags | quickly browse through all the tags that I have added |
* * * |
student | add tasks for each tag | remind myself of all the tasks that are under those tags. |
* * * |
student | delete tasks for each tag | remove the tasks that are no longer required for that module |
* * * |
student with many project groups | add the meeting platform links to each tag | conveniently contact the team or initiate a team meeting |
* * * |
student with many project groups | delete the meeting platform link for each tag | remove the link if it has been added wrongly |
* * |
student | clear all completed tasks under each tag | remove the tasks that are no longer required |
* * |
user | update a tag (name) | correct the misspelled tag name |
* * |
user | sort the tags by its tag name | view tags in alphabetical order |
* * |
user with many contacts | sort the contacts by name | view contacts in alphabetical order |
* * |
student | mark the status of a particular task as done | keep track of the different task progress under that tag. |
* * |
student with many project groups | add remarks on the tag | find module-related information from the tag |
* * |
student with many project groups | delete the comments on the tag | |
* |
long-time user | archive old tags | keep my contacts up to date |
* |
long-time user | unarchive old tags | conveniently use the same old tag containing the same contact |
* |
power user | create shortcuts for existing commands | type faster in my preferred way for certain commands |
* |
power user | import and export person list to another device | save time compiling the person list |
C: Use Cases
(For all use cases below, the System is the Projact
and the Actor is the user
, unless specified otherwise)
Use case: UC01 - Delete a person
MSS
- User requests to list persons
- Projact shows a list of persons
- User requests to delete a specific person in the list
-
Projact deletes the person
Use case ends.
Extensions
-
2a. The list containing all the persons is empty.
Use case ends.
-
3a. The given index is invalid.
-
3a1. Projact shows an error message.
Use case resumes at step 2.
-
Use case: UC02 - List all tags
MSS
- User requests to list tags
-
Projact shows a list of tags
Use case ends.
Extensions
-
2a. The list containing all the tags is empty.
Use case ends.
Use case: UC03 - Create a Tag
MSS
- User requests to create a tag
-
Projact adds the tag.
Use case ends.
Extensions
-
2a. The tag already exists.
- 2a1. Projact shows an error message.
Use case ends.
Use case: UC04 - Delete a tag
MSS
- User requests to list tags
- Projact shows a list of tags
- User requests to delete a specific tag in the list
-
Projact deletes the tag
Use case ends.
Extensions
-
2a. The list containing all the tags is empty.
Use case ends.
-
3a. The given index is invalid.
-
3a1. Projact shows an error message.
Use case resumes at step 2.
-
Use case: UC05 - Find a tag
MSS
- User searches for tag(s) by keyword(s)
-
Projact displays a list of matching tags
Use case ends.
Extensions
-
2a. The list containing all the tags is empty.
Use case ends.
-
2b. There are no tags that match the keyword(s)
Use case ends.
-
2c. User did not specify any keywords.
-
2c1. Projact shows an error message.
Use case resumes at step 1.
-
Use case: UC06 - Edit tag
MSS
- User requests to list tags (UC02)
- User requests to edit a specific tag in the list
-
Projact edits the tag
Use case ends.
Extensions
-
1a. The list containing all the tags is empty.
Use case ends.
-
2a. The given index is invalid.
-
2a1. Projact shows an error message.
Use case resumes at step 2.
-
-
2b. The new tag name is missing.
-
2b1. Projact shows an error message.
Use case resumes at step 2.
-
Use case: UC07 - Add a link to a tag
MSS
- User requests to list tags (UC02)
- User requests to add a link to a tag in the list.
-
Projact adds the link to the tag.
Use case ends.
Extensions
-
1a. The list containing all the tags is empty.
Use case ends.
-
2a. The given index is invalid.
-
2a1. Projact shows an error message.
Use case resumes at step 2.
-
-
2b. The tag already has a link.
-
2b1. Projact shows an error message.
Use case resumes at step 2.
-
-
2c. The input link is not valid.
-
2b1. Projact shows an error message.
Use case resumes at step 2.
-
Use case: UC08 - Mark a task as done
MSS
- User requests to list tags (UC02)
- User requests to mark a particular task from a tag in the list as done.
-
Projact changes the status of the task to ‘done’.
Use case ends.
Extensions
-
1a. The list containing all the tags is empty.
Use case ends.
-
2a. The given index is invalid.
-
2a1. Projact shows an error message.
Use case resumes at step 2.
-
-
2b. The targeted task has been completed.
-
2b1. Projact shows an error message.
Use case resumes at step 2.
-
Use case: UC09 - Clear completed tasks
MSS
- User requests to list tags (UC02)
- User requests to delete all completed tasks from a tag in the list
-
Projact deletes all completed tasks from the tag
Use case ends.
Extensions
-
1a. The list is empty.
Use case ends.
-
2a. The given index is invalid.
-
2a1. Projact shows an error message.
Use case resumes at step 2.
-
-
2b. The tag has no completed tasks.
-
2b1. Projact shows an error message.
Use case resumes at step 2.
-
D: Non-Functional Requirements
- Should work on any mainstream OS as long as it has Java
11
or above installed. - Should be able to hold up to 1000 persons without a noticeable sluggishness in performance for typical usage.
- A user with above average typing speed for regular English text (i.e. not code, not system admin commands) should be able to accomplish most of the tasks faster using commands than using the mouse.
- The system should work on both 32-bit and 64-bit environments.
- The software should work without requiring an installer.
- The software should not depend on any of the team member’s own remote server.
- The product should not use images that would result in copyright infringement.
- The team should give credit for any reused code.
- The software should not depend on any third-party frameworks/libraries that are not approved by the CS2103T teaching team.
- The software should obey Java checkstyle rules.
- The system should respond within two seconds.
- The product is not required to handle the communication between the user and those in the user’s projact.
- The product should be customised to target NUS Computing students.
- The system should be usable by a novice who has never used a command line interface before.
- The product should be targeting users who can type fast and prefer typing over other means of input.
- The product should be for a single user i.e. (not a multi-user product).
- The data should be stored locally and should be in a human editable text file.
- The data should not be stored using a Database Management System (DBMS).
- The data should be updated as the product is being used (update with every command).
- The user’s data should not be accessible to anyone who does not have access to the user’s local files.
- The user’s data should not be accessible by developers of the product.
E: Glossary
- Contact: A person’s information, which includes the name, phone number, email address and Telegram handle of the person
- Tag: A label to group and/or distinguish different contacts. Examples: Relationship with person (friend, family, etc), common module taken (MA1101R, CS2101, etc)
- Mainstream OS: Windows, Linux, Unix, OS-X
F: Instructions for manual testing
Given below are instructions to test the app manually.

Launch and shutdown
-
Initial launch
-
Download the jar file and copy into an empty folder
-
Double-click the jar file Expected: Shows the GUI with a set of sample contacts. The window size may not be optimum.
-
-
Saving window preferences
-
Resize the window to an optimum size. Move the window to a different location. Close the window.
-
Re-launch the app by double-clicking the jar file.
Expected: The most recent window size and location is retained.
-
Contact Commands
Adds a person
-
Adding a person while all persons are being shown
-
Prerequisites: List all persons using the
list
command. Multiple persons in the list. -
Test case:
add n/Jane p/98765432 e/jane@gmail.com ta/jane_tan t/cs2103
Expected: A new contact is added to the end of the persons list with the contact details set to the respective user inputs. Contact details are shown in the status message. -
Test case:
add n/Jane p/98765432 e/jane@gmail.com
Expected: No person is added. Invalid command format error shown in the status message due to missingta/TELEGRAM_ADDRESS
field. -
Test case:
add n/Jane p/98881999 e/janelim@gmail.com ta/jane_lim t/cs2103
Expected: No person is added. Duplicate person error shown in the status message due to existing contact having the exact same name.
-
Edits a person
-
Editing a person while all persons are being shown
-
Prerequisites: List all persons using the
list
command. Multiple persons in the list. -
Test case:
edit 1 n/James Lee e/jameslee@example.com
Expected: Contact details of the first contact in the list is set to the respective user inputs. Updated contact details are shown in the status message. -
Test case:
edit 0 n/James Lee
Expected: No person is edited. Invalid command format error shown in the status message due to non-positive index indicated. -
Test case:
edit 1
Expected: No person is edited. Error message stating that there should be at least one field provided in shown in the status message. -
Test case:
edit x
(where x is larger than the list size)
Expected: No person is edited. Error message stating that index is invalid is shown in the status message.
-
Deletes a person
-
Deleting a person while all persons are being shown
-
Prerequisites: List all persons using the
list
command. Multiple persons in the list. -
Test case:
delete 1
Expected: First contact is deleted from the list. Details of the deleted contact shown in the status message. -
Test case:
delete 0
Expected: No person is deleted. Error details shown in the status message. Status bar remains the same. -
Other incorrect delete commands to try:
delete
,delete x
,...
(where x is larger than the list size)
Expected: Similar to previous.
-
Finds a person
-
Finding a person while all persons are being shown
-
Prerequisites: List all persons using the
list
command. Multiple persons in the list. For the following test cases, assume that the list contains the contacts: Jane, John, JanE. -
Test case:
find john
Expected: The contact John and his contact details is listed. The number of contacts listed is shown in the status message. -
Test case:
find jane
Expected: The contacts Jane and JanE are listed. The number of contacts listed is shown in the status message. -
Test case:
find james
Expected: No contacts are listed. The number of contacts listed is shown in the status message. -
Test case:
find
Expected: Invalid command format error is shown in the status message due to missingKEYWORD
field.
-
Tag Commands
The manual testing of tag commands such as tagadd
, tagedit
, tagdelete
, tagfind
can be done in a similar to the contact commands. However, instead of using list
to list all tags,
taglist
should be used instead.
Link Commands
Adds a link
-
Adding a link to a tag while all tags are being shown
-
Prerequisites: List all tags using the
taglist
command. For step 2, assume the first tag in the list has no pre-existing link. -
Test case:
linkadd 1 l/http://zoom.com/
Expected: The link is added to the first tag in the tag list. The link is shown in the status message. -
Test case:
linkadd 1 l/http://google.com/
Expected: No link is added. Error message stating there is already a link is shown in the status message. -
Test case:
linkadd 2
Expected: No link is added. Error message stating that there should be a link is shown in the status message. -
Test case:
linkadd 2 l/http
Expected: No link is added. Error message stating the correct link format is shown in the status message.
-
Deletes a link
-
Deleting a link from a tag while all the tags are being shown
-
Prerequisites: List all tags using the
taglist
command. For the following test cases, assume that only the first tag has a link. -
Test case:
linkdelete 1
Expected: Link is deleted from the first tag in the list. Details of deleted link is shown in the status message. -
Test case:
linkdelete 2
Expected: No link is deleted. Error message stating that the tag does not have a link is shown in the status message. -
Test case:
linkdelete 0
Expected: No link is deleted. Invalid command format error is shown in the status message due to non-positive index.
-
Task Commands
Adds a task
-
Adding a task to a tag while all tags are being shown
-
Prerequisites: List all tags using the
taglist
command. -
Test case:
taskadd 1 task/submit reflection
Expected: Task is added to the first tag in the list, with a cross beside it to indicate that the task is incomplete. Task details are shown in the status message. -
Test case:
taskadd 1
Expected: No task is added. Invalid command format error shown in the status message due to missingtask/TASK_NAME
field. -
Test case:
taskadd 5 task/peer review
Expected: No task is added. Error message stating that index is invalid is shown in the status message.
-
Marks a task as done
-
Marking a task as done while all tags are being shown
-
Prerequisites: List all tags using the
taglist
command. For the following test cases, assume that the first tag has 1 task, while the other tags have none. -
Test case:
taskdone 1 a
Expected: The first task in the first tag in the list is marked as done. Cross changes to a tick to indicate that the task is completed. Task details are shown in the status message. -
Test case:
taskdone 1 a
(repeating the same command)
Expected: Message stating that the task has already been completed is shown in the status message. -
Test case:
taskdone 1 b
Expected: No task is marked as done. Error message stating that the task index is invalid is shown in the status message. -
Test case:
taskdone 2 a
Expected: No task is marked as done. Error message stating that there are no tasks under the indicated tag is shown in the status message.
-
Deletes a task
-
Deleting a task from a tag while all the tags are being shown
-
Prerequisites: List all tags using the
taglist
command. For the following test cases, assume that the first tag has 1 task, while the other tags have none. -
Test case:
taskdelete 1 a
Expected: The first task in the first tag in the list is deleted. Details of deleted task is shown in the status message. -
Test case:
taskdelete 1 a
(repeating the same command)
Expected: No task is deleted. Error message stating that there are no tasks under the indicated tag is shown in the status message. -
Test case:
taskdelete 2 a
Expected: No task is deleted. Error message stating that there are no tasks under the indicated tag is shown in the status message.
-
Clears completed tasks
-
Clearing the completed tasks from a tag while all the tags are being shown
-
Prerequisites: List all tags using the
taglist
command. For the following test cases, assume that the first tag has 2 completed tasks, while the other tags have none. -
Test case:
taskclear 1
Expected: All the completed tasks in the first tag in the list are deleted. Success message is shown in the status message. -
Test case:
taskclear 1
(repeating the same command)
Expected: Error message stating that there are no completed tasks under the indicated tag is shown in the status message. -
Test case:
taskdelete 2 a
Expected: Error message stating that there are no completed tasks under the indicated tag is shown in the status message.
-
Saves data
-
Dealing with missing/corrupted data files
-
If the data file is missing, the application will launch a window that is populated with the sample data. User can use the
clear
command to get an empty projact window. Expected: Window with sample data -
If the data file is corrupted, users should delete the
projact.json
file in data folder. Relaunch the jar file again. Expected: Window with sample data
-